How to Create User Registration and Login with PHP and MySQL
Do you know how to create Registration and Login with PHP and MySQL ? if you are a PHP beginner you wish to learn how to create user registration with PHP and MySQL
so here I am writing a script to create a user login with PHP and MySQL
Here i will explain all the steps to create Registration and Login with PHP and if you are confused with the list of codes , dont worry i will add my explainer video and complete source code completely for free.
STEP: 1 CREATE DATABASE (run in phpmyadmin)
CREATE TABLE users ( id INT NOT NULL PRIMARY KEY AUTO_INCREMENT, username VARCHAR(50) NOT NULL UNIQUE, password VARCHAR(255) NOT NULL, created_at DATETIME DEFAULT CURRENT_TIMESTAMP );
STEP 2: CREATING CONFIG FILE (config.php)
<?php error_reporting(0); define('DB_SERVER', 'localhost'); define('DB_USERNAME', 'root'); define('DB_PASSWORD', ''); define('DB_NAME', 'demo'); $link = mysqli_connect(DB_SERVER, DB_USERNAME, DB_PASSWORD, DB_NAME); if($link === false){ die("ERROR: Could not connect. " . mysqli_connect_error()); } ?>
STEP 3 : CREATING REGISTRATION FORM (signup.php)
<html> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-BmbxuPwQa2lc/FVzBcNJ7UAyJxM6wuqIj61tLrc4wSX0szH/Ev+nYRRuWlolflfl" crossorigin="anonymous"> <div class="container"> <div class="col-md-6"> <h2> Signup Form </h2> <form method="post" action="createaccount.php"> <input type="text" name="username" class="form-control" placeholder="Username"> <input type="password" name="password" class="form-control" placeholder="Password"> <input type="submit" value="Register"> </form> </div> </html>
Create a file called createaccount.php and adding account creation PHP codes (createaccount.php)
<?php session_start(); require_once "config.php"; if($_SERVER["REQUEST_METHOD"] == "POST"){ $username = $_POST["username"]; $password = $_POST["password"]; // hashing the password $password = md5($password ); //checking username already exists $checking = mysqli_query($link,"SELECT * FROM users WHERE username='$username'"); $checkcount = mysqli_num_rows($checking); if($checkcount!=0){ echo "Username Already exists, Please try another one"; } else { mysqli_query($link,"INSERT INTO `users` (`username`, `password`) VALUES ('$username', '$password')"); // getting user id $userid = mysqli_insert_id($link); //creating session $_SESSION["userid"] = $userid; header("location:dashboard.php"); } }
STEP 4: DASHBOARD (dashboard.php)
<?php session_start(); require_once "config.php"; $userid = $_SESSION["userid"]; if($userid!=NULL){ $getdata = mysqli_query($link,"SELECT * FROM users WHERE id='$userid'"); $userdata = mysqli_fetch_array($getdata); ?> <html> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-BmbxuPwQa2lc/FVzBcNJ7UAyJxM6wuqIj61tLrc4wSX0szH/Ev+nYRRuWlolflfl" crossorigin="anonymous"> <div class="container"> <h2>Dashboard </h2> <h3> Welcome <?php echo $userdata['username']; ?> ! </h3> <p> Registred at <?php echo $userdata['created_at']; ?> ! </p> <h6> <a href="logout.php"> Logout </a> </h6> </div> </html> <?php } else { echo "You are not loged in"; } ?>
STEP 5: LOGIN PAGE (login.php)
<html> <link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-BmbxuPwQa2lc/FVzBcNJ7UAyJxM6wuqIj61tLrc4wSX0szH/Ev+nYRRuWlolflfl" crossorigin="anonymous"> <div class="container"> <div class="col-md-6"> <h2> Login Form </h2> <form method="post" action="logincheck.php"> <input type="text" name="username" class="form-control" placeholder="Username"> <input type="password" name="password" class="form-control" placeholder="Password"> <input type="submit" value="Login"> </form> </div> </html>
(logincheck.php) file
<?php session_start(); require_once "config.php"; if($_SERVER["REQUEST_METHOD"] == "POST"){ $username = $_POST["username"]; $password = $_POST["password"]; // hashing the password $password = md5($password ); //checking username already exists if($username!=NULL){ $checking = mysqli_query($link,"SELECT * FROM users WHERE `username`='$username' AND `password`='$password'"); // getting user id $userdata = mysqli_fetch_array($checking); $userid = $userdata['id']; if($userid!=NULL) { //creating session $_SESSION["userid"] = $userid; header("location:dashboard.php"); } else { header("location:login.php?error=1"); } } else { } }
STEP 6 : LOGOUT (logout.php)
<?php session_start(); $_SESSION = array(); session_destroy(); header("location: login.php"); exit; ?>
checkout the video for a detailed explanation
Download Source Code :
Hope this helpful for you.
You can check out our awesome PHP tutorials, it will very helpful if you are a beginner
What tools i have to use to create Registration and Login with PHP
Where the datas will be saved
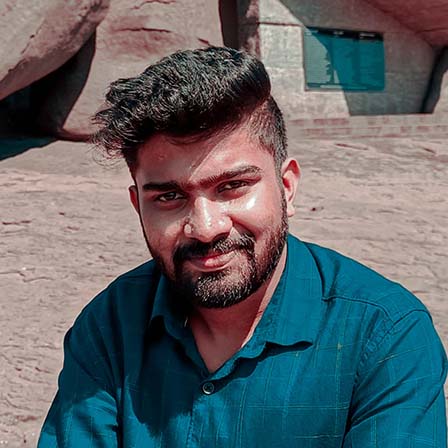
Share with your friends:
Drag and Drop with Swapy and PHP
Hello , You might had issues with drag and drop options in your dashboard. here i found a new javascript […]
September 17, 2024
How To Generate A PDF from HTML in Laravel 11
Hello , I was trying to generate a PDF payment receipt for my SAAS application and when i search for […]
June 22, 2024
How to get the next value of an array and loop it in the array in PHP
Do you know How to get the next value of an array and loop it in the array in PHP […]
July 28, 2023
New Open Source CRM for project Management and Invoicing
I’m excited to announce the launch of my new open source project: Gmax CRM. an invoicing and project management tool […]
December 31, 2022
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now
Leave a Reply