How to add Google and GitHub auth into a laravel application
Do you know How to add Google and GitHub auth into a laravel application ?
It’s very easy to implement Google and GitHub authentication into your Laravel application. we are using the Laravel socialite package to do that. I will show you how easy to make that happen.
I hope you are familiar with Laravel so I am not covering the basics.
First, we have to install Laravel socialite package in our application
composer require laravel/socialite
Now we have to configure it inside our
config/services.php
file.
Add these codes to your services.php file
'github' => [ 'client_id' => env('GITHUB_CLIENT_ID'), 'client_secret' => env('GITHUB_CLIENT_SECRET'), 'redirect' => '/auth/github/callback', ], 'google' => [ 'client_id' => env('GOOGLE_CLIENT_ID'), 'client_secret' => env('GOOGLE_CLIENT_SECRET'), 'redirect' => '/auth/google/callback', ],
Now we have to create a controller
php artisan make:controller socialauthcontroller
Open the controller and add these functions
// enter controller code
use Laravel\Socialite\Facades\Socialite; use App\Models\User; use Illuminate\Support\Facades\Auth; use Illuminate\Support\Str; class socialauthcontroller extends Controller { public function githubredirect(Request $request) { return Socialite::driver('github')->redirect(); } public function githubcallaback(Request $request) { $userdata = Socialite::driver('github')->user(); //check login $user = User::where('email', $userdata->email)->where('auth_type','github')->first(); if($user) { Auth::login($user); return redirect('/dashboard'); } else { //do register $uuid = Str::uuid()->toString(); $user =new User(); $user->name =$userdata->name; $user->email =$userdata->email; $user->password =Hash::make($uuid.now()); $user->auth_type ='github'; $user->save(); Auth::login($user); return redirect('/dashboard'); } } public function googleredirect(Request $request) { return Socialite::driver('google')->redirect(); } public function googlecallaback(Request $request) { $userdata = Socialite::driver('google')->user(); //check login $user = User::where('email', $userdata->email)->where('auth_type','google')->first(); if($user) { Auth::login($user); return redirect('/dashboard'); } else { //do register $uuid = Str::uuid()->toString(); $user =new User(); $user->name =$userdata->name; $user->email =$userdata->email; $user->password =Hash::make($uuid.now()); $user->auth_type ='google'; $user->save(); Auth::login($user); return redirect('/dashboard'); } } }
now edit the .env file and add credentials. if you don’t know how to get google and GitHub client id and secret please check the video i made a complete detailed explanation about this topic.
GITHUB_CLIENT_ID=xxxxxxxxxxxxxxxxxxxxxxx GITHUB_CLIENT_SECRET=xxxxxxxxxxxxxxxxxxxxxxxxxxxx GOOGLE_CLIENT_ID=xxxxxxxxxxxxxxxxxxxxxxxxx GOOGLE_CLIENT_SECRET=xxxxxxxxxxxxxxxxxxxxxxxxxxxxx
Now what … we have to create the routes
use App\Http\Controllers\socialauthcontroller; use Laravel\Socialite\Facades\Socialite; Route::get('/auth/github/redirect', [socialauthcontroller::class, 'githubredirect'])->name('githubredirect'); Route::get('/auth/github/callback', [socialauthcontroller::class, 'githubcallaback'])->name('githubcallaback'); Route::get('/auth/google/redirect', [socialauthcontroller::class, 'googleredirect'])->name('googleredirect'); Route::get('/auth/google/callback', [socialauthcontroller::class, 'googlecallaback'])->name('googlecallaback');
Okay that’s done. now we have to test it. if that working and you are getting your profile information now we can move on to create a use with that .
Now go to yourwebsite.com/auth/github/redirectÂ
to check github login
and go to yourwebsite.com/auth/google/redirectÂ
to check google login
Okay seems nice ..
You could able to log in with google and Github now.
Conclusion:
Its very easy to make social authentication into websites. and anybody can able to do within minutes. In this tutorial you learned to install the socialite package, add config data, add API keys into env file, create a controller and create users from API output etc. hope this helps to make your web application more powerful.
Explainer Video About adding Google and GitHub auth into a Laravel application
Checkout more Awesome Laravel Tutorials Here
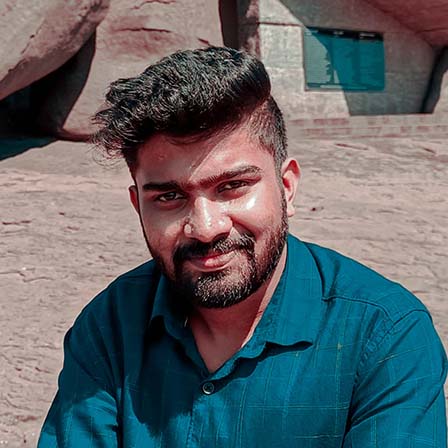
Share with your friends:
How To Generate A PDF from HTML in Laravel 11
Hello , I was trying to generate a PDF payment receipt for my SAAS application and when i search for […]
June 22, 2024
How to create Laravel Flash Messages
Laravel flash messages are a convenient way to display one-time notifications to the user after a form submission or other […]
December 22, 2022
How to make Custom Artisan Command in Laravel
Custom Artisan commands are a useful feature of Laravel that allow you to define your own command-line commands for tasks […]
December 22, 2022
Tips for Laravel migrations
Laravel migrations are a powerful tool for managing and modifying your database schema in a structured and organized way. Here […]
December 22, 2022
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now