HTML from to Email using SMTP in PHP with Source code
Hello Everyone,
In this tutorial, I am going to teach you how to send an email from an HTML form to your email id using PHP and SMTP. 💡
Here we are using PHPmailer to send emails using SMTP. in the source code you can download all the code including the library.
To test SMTP I used a website that help us to test email servers which is mailtrap.io
You can signup in it for free if you want to test with that.
If you are using composer you can download it by using this command composer require phpmailer/phpmailer
So first we have to make an HTML form (looks like our contact form)
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Send Mail</title> </head> <body> <h3> Send Email using SMTP </h3> <form action="mail.php" method="post"> <label for="name">Your Name</label> <input type="text" name="name" placeholder="Enter Name"> <br> <label for="lname">Email</label> <input type="email" name="email" placeholder="Enter Email"> <br> <label for="message">Message</label> <textarea name="message" placeholder="Enter Message" rows="3"> </textarea> <br> <button type="submit"> Send Email </button> </form> </body> </html>
Once we made our contact form we have to create another file called mail.php (in our case, because we set the form action to mail.php, you can name it whatever you want)
so Creating our mail.php file
<?php //Import PHPMailer classes into the global namespace //These must be at the top of your script, not inside a function use PHPMailer\PHPMailer\PHPMailer; use PHPMailer\PHPMailer\SMTP; use PHPMailer\PHPMailer\Exception; //Load Composer's autoloader require 'vendor/autoload.php'; //get data from form $name = $_POST['name']; $email = $_POST['email']; $message = $_POST['message']; // preparing mail content $messagecontent ="Name = ". $name . "<br>Email = " . $email . "<br>Message =" . $message; //Create an instance; passing `true` enables exceptions $mail = new PHPMailer(true); try { //Server settings //$mail->SMTPDebug = SMTP::DEBUG_SERVER; //Enable verbose debug output $mail->isSMTP(); //Send using SMTP $mail->Host = 'smtp.mailtrap.io'; //Set the SMTP server to send through $mail->SMTPAuth = true; //Enable SMTP authentication $mail->Username = 'username_here'; //SMTP username $mail->Password = 'password_here'; //SMTP password // $mail->SMTPSecure = PHPMailer::ENCRYPTION_SMTPS; //Enable implicit TLS encryption $mail->Port = 2525; //TCP port to connect to; use 587 if you have set `SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS` //Recipients $mail->setFrom('[email protected]', 'Mailer'); $mail->addAddress('[email protected]', 'Joe User'); //Add a recipient $mail->addAddress('[email protected]'); //Name is optional $mail->addReplyTo('[email protected]', 'Information'); $mail->addCC('[email protected]'); $mail->addBCC('[email protected]'); //Attachments //$mail->addAttachment('/var/tmp/file.tar.gz'); //Add attachments // $mail->addAttachment('photo.jpeg', 'photo.jpeg'); //Optional name //Content $mail->isHTML(true); //Set email format to HTML $mail->Subject = 'Here is the subject'; $mail->Body = $messagecontent; $mail->send(); echo 'Message has been sent'; } catch (Exception $e) { // echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}"; }
Yes, you can use this code to send emails. in this code we are getting the input values from the form and storing them in different variables, then we make a string from it and storing in a single variable.
then we are pushing that data to the email address. You can download the package files from PHPmailer Repository or you can get this complete source code from here.
Hope this helps . Happy Coding  😀
Download Source Code :Â
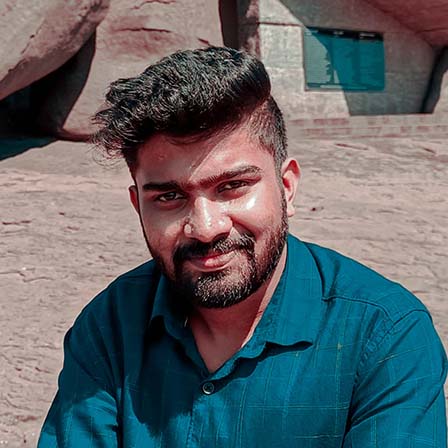
Share with your friends:
Drag and Drop with Swapy and PHP
Hello , You might had issues with drag and drop options in your dashboard. here i found a new javascript […]
September 17, 2024
How To Generate A PDF from HTML in Laravel 11
Hello , I was trying to generate a PDF payment receipt for my SAAS application and when i search for […]
June 22, 2024
How to get the next value of an array and loop it in the array in PHP
Do you know How to get the next value of an array and loop it in the array in PHP […]
July 28, 2023
New Open Source CRM for project Management and Invoicing
I’m excited to announce the launch of my new open source project: Gmax CRM. an invoicing and project management tool […]
December 31, 2022
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now