Handling File Uploads in Laravel
**Handling File Uploads in Laravel: A Complete Guide**
Welcome back to our blog! Today, we are diving into a common task in web development – handling file uploads in Laravel. Whether you are building a simple contact form or a full-fledged application with user profile pictures, understanding how to handle file uploads is crucial. Laravel makes this process simple and efficient with its built-in features and powerful tools. In this post, we will walk you through the process of handling file uploads in Laravel step by step with code snippets and explanations.
**Setting Up a Form for File Upload**
The first step in handling file uploads is creating a form that allows users to submit files. Let’s start by creating a simple form in a Laravel view file. Here is an example of a basic form for file upload:
html
<form method="POST" action="{{ route('upload.file') }}" enctype="multipart/form-data">
@csrf
<input type="file" name="file">
<button type="submit">Upload</button>
</form>
In the form above, we have set the form method to POST and specified the action to be handled by a route named ‘upload.file’. The important part here is to add the `enctype=”multipart/form-data”` attribute to the form tag. This attribute is necessary for handling file uploads in Laravel.
**Processing File Uploads in a Controller**
Next, let’s create a controller method that will handle the file upload. In your controller, you can access the uploaded file using the `Request` object and store it in a secure location on your server. Here is an example of a controller method for handling file uploads:
php
public function uploadFile(Request $request)
{
if ($request->hasFile('file')) {
$file = $request->file('file');
$fileName = time() . '_' . $file->getClientOriginalName();
$file->storeAs('uploads', $fileName);
// Additional logic such as saving file information to a database can be added here
return "File uploaded successfully!";
}
return "No file uploaded.";
}
In the controller method above, we first check if a file has been uploaded. If a file is present, we retrieve the file object using `$request->file(‘file’)`. We generate a unique file name using the current timestamp and the original file name and then store the file in the ‘uploads’ directory using the `storeAs` method. You can customize the file storage location based on your requirements.
**Validating File Uploads**
File uploads are often subject to validation to ensure the uploaded files meet certain criteria such as file type, size, and dimensions. Laravel provides convenient validation rules for file uploads. Here is an example of validating file uploads in a Laravel controller method:
php
$request->validate([
'file' => 'required|file|mimes:jpeg,png|max:2048',
]);
In the example above, we are validating the ‘file’ input to be required, a file type, either JPEG or PNG, and a maximum file size of 2MB. You can customize these validation rules based on your project requirements.
**Summary**
Handling file uploads in Laravel is a common task in web development, but with Laravel’s powerful features, the process becomes straightforward and efficient. In this post, we have covered the essentials of handling file uploads in Laravel, from setting up a form for file upload to processing file uploads in a controller and validating file uploads. By following these steps and best practices, you can create robust file upload functionality in your Laravel applications. Stay tuned for more Laravel tips and tricks on our blog!
Post Your Questions on our forum
Post a question on Forum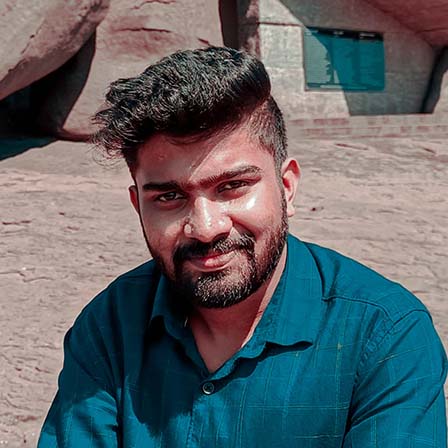
Share with your friends:
How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this […]
April 3, 2024
How to integrate Razorpay API in Laravel
Integrating payment gateways into web applications has become an essential part of e-commerce websites. In this tutorial, we will discuss […]
April 3, 2024
Laravel 11 Ajax CRUD Operation Tutorial Example
**Mastering CRUD Operations with Laravel 11 Ajax: A Comprehensive Tutorial** In the world of web development, interaction between the front-end […]
April 3, 2024
Login as Client in Laravel – Login with user id
**Unlock the Power of Laravel with Login as Client – Login with User ID** Laravel, the popular PHP framework, offers […]
April 3, 2024
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now