How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this tutorial, we will guide you through the step-by-step process of integrating Paypal API in Laravel. By the end of this blog post, you will be able to securely accept payments using Paypal in your Laravel application.
**Setting Up Paypal API Credentials**
The first step to integrate Paypal API in Laravel is to obtain API credentials from Paypal. You will need to create a Paypal developer account and generate API credentials such as Client ID and Secret Key. These credentials will be used to authenticate your Laravel application with Paypal’s API.
**Installing Paypal SDK**
Next, you need to install the Paypal SDK package in your Laravel project. You can do this using Composer by running the following command in your terminal:
bash
composer require paypal/rest-api-sdk-php
Once the package is installed, you can start using Paypal SDK in your Laravel application to interact with Paypal’s API.
**Creating Paypal Payment Gateway**
To create a Paypal payment gateway in your Laravel application, you can use the Paypal SDK to set up a payment request with the required details such as amount, currency, and redirect URLs. Here is an example code snippet to create a Paypal payment gateway in Laravel:
php
use PayPal\Api\Payer;
use PayPal\Api\Amount;
use PayPal\Api\Transaction;
use PayPal\Api\RedirectUrls;
use PayPal\Api\Payment;
$payer = new Payer();
$payer->setPaymentMethod('paypal');
$amount = new Amount();
$amount->setTotal('10.00');
$amount->setCurrency('USD');
$transaction = new Transaction();
$transaction->setAmount($amount);
$redirectUrls = new RedirectUrls();
$redirectUrls->setReturnUrl('http://yourwebsite.com/return')
->setCancelUrl('http://yourwebsite.com/cancel');
$payment = new Payment();
$payment->setIntent('sale')
->setPayer($payer)
->setTransactions([$transaction])
->setRedirectUrls($redirectUrls);
$payment->create($apiContext); // $apiContext is your Paypal API credentials
**Handling Payment Response**
Once the Paypal payment is processed, Paypal will redirect the user back to your Laravel application with a payment response. You can handle this response by verifying the payment status and completing the transaction in your Laravel controller. Here is an example code snippet to handle payment response in Laravel:
php
use PayPal\Api\Payment;
$paymentId = request('paymentId');
$payment = Payment::get($paymentId, $apiContext); // $apiContext is your Paypal API credentials
if ($payment->getState() == 'approved') {
// Payment is successful, complete the transaction
// Add your business logic here
} else {
// Payment is not successful, handle accordingly
// Redirect user to payment failure page
}
**Conclusion**
Integrating Paypal API in Laravel is a straightforward process that allows you to securely accept payments in your application. By following the steps outlined in this tutorial, you can easily set up a Paypal payment gateway and handle payment responses in your Laravel project. Start integrating Paypal API in Laravel today and enhance your payment processing capabilities!
Post Your Questions on our forum
Post a question on Forum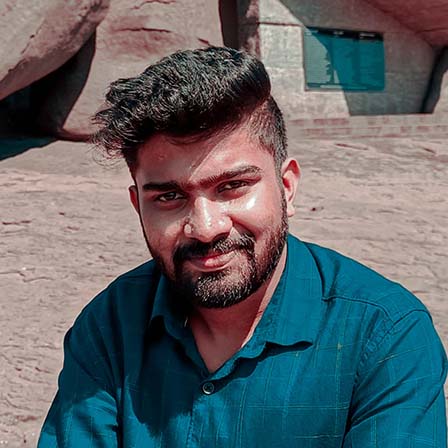
Share with your friends:
How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this […]
April 3, 2024
How to integrate Razorpay API in Laravel
Integrating payment gateways into web applications has become an essential part of e-commerce websites. In this tutorial, we will discuss […]
April 3, 2024
Laravel 11 Ajax CRUD Operation Tutorial Example
**Mastering CRUD Operations with Laravel 11 Ajax: A Comprehensive Tutorial** In the world of web development, interaction between the front-end […]
April 3, 2024
Login as Client in Laravel – Login with user id
**Unlock the Power of Laravel with Login as Client – Login with User ID** Laravel, the popular PHP framework, offers […]
April 3, 2024
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now