Laravel 11 CRUD Application Example Tutorial
Are you looking to enhance your Laravel skills and learn how to build a CRUD application using Laravel 11? Look no further! In this tutorial, we will guide you through creating a simple CRUD application with Laravel 11, covering the basics of creating, reading, updating, and deleting data.
### **Setting Up Your Laravel Project**
Before we dive into building our CRUD application, we first need to set up our Laravel project. If you haven’t already installed Laravel, you can do so by running the following command in your terminal:
bash
composer create-project --prefer-dist laravel/laravel crud-app
Next, navigate to your project directory:
bash
cd crud-app
To generate a model and migration for our CRUD application, run the following command:
bash
php artisan make:model Item -m
This command will create a model named Item along with a migration file, which we will use to define our database schema.
### **Creating the Database Schema**
Open the migration file created for the Item model (located in `database/migrations`) and define the schema for the items table. Here is an example of what the migration file might look like:
php
public function up()
{
Schema::create('items', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->timestamps();
});
}
After defining the schema, run the migration command to create the items table in your database:
bash
php artisan migrate
### **Creating Routes**
Next, let’s create routes for our CRUD operations. Open the `routes/web.php` file in your project and define the routes for creating, reading, updating, and deleting items:
php
Route::get('/items', 'App\Http\Controllers\ItemController@index');
Route::post('/items', 'App\Http\Controllers\ItemController@store');
Route::get('/items/{id}', 'App\Http\Controllers\ItemController@show');
Route::put('/items/{id}', 'App\Http\Controllers\ItemController@update');
Route::delete('/items/{id}', 'App\Http\Controllers\ItemController@destroy');
### **Creating the Controller**
Now, let’s create the controller that will handle our CRUD operations. Generate a new controller named `ItemController` using the following command:
bash
php artisan make:controller ItemController
In the `ItemController`, define the methods for the CRUD operations:
php
public function index()
{
// Retrieve all items
}
public function store(Request $request)
{
// Create a new item
}
public function show($id)
{
// Retrieve a specific item
}
public function update(Request $request, $id)
{
// Update an existing item
}
public function destroy($id)
{
// Delete an item
}
### **Creating Views**
Finally, let’s create the views to display our CRUD operations. Create the necessary Blade templates to create, read, update, and delete items in the `resources/views` directory.
### **Conclusion**
Congratulations! You have successfully set up a basic CRUD application using Laravel 11. This tutorial covered the essential steps to create, read, update, and delete data in your Laravel application. Building CRUD functionality is a fundamental aspect of web development, and mastering it will enhance your skills as a Laravel developer.
Feel free to experiment with the CRUD operations and customize the application further to suit your needs. Laravel provides a robust framework for building web applications, and understanding CRUD operations is a crucial step in becoming proficient in Laravel development.
Happy coding!
Post Your Questions on our forum
Post a question on Forum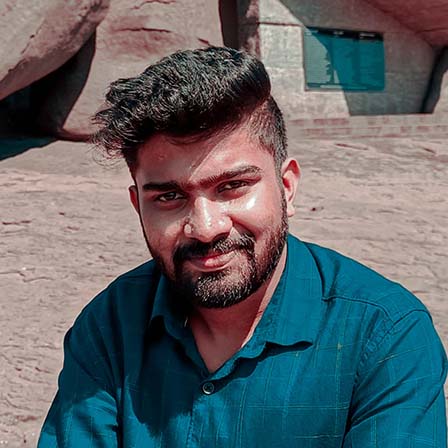
Share with your friends:
How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this […]
April 3, 2024
How to integrate Razorpay API in Laravel
Integrating payment gateways into web applications has become an essential part of e-commerce websites. In this tutorial, we will discuss […]
April 3, 2024
Laravel 11 Ajax CRUD Operation Tutorial Example
**Mastering CRUD Operations with Laravel 11 Ajax: A Comprehensive Tutorial** In the world of web development, interaction between the front-end […]
April 3, 2024
Login as Client in Laravel – Login with user id
**Unlock the Power of Laravel with Login as Client – Login with User ID** Laravel, the popular PHP framework, offers […]
April 3, 2024
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now