Laravel 11 RESTful API Development
**Laravel 11 RESTful API Development: A Comprehensive Guide**
In today’s digital world, building robust APIs is a crucial part of web development. Laravel, a popular PHP framework, offers a powerful toolset for creating RESTful APIs quickly and efficiently. In this blog post, we’ll delve into Laravel 11 RESTful API development, covering key concepts and providing code examples to help you get started.
**What is a RESTful API?**
REST (Representational State Transfer) is an architectural style for designing networked applications. RESTful APIs adhere to REST principles, allowing clients to interact with server resources through predefined operations (HTTP methods) such as GET, POST, PUT, and DELETE. This approach promotes scalability, flexibility, and simplicity in API design.
**Setting up Laravel 11 for API Development**
To begin building a RESTful API with Laravel 11, you first need to set up a new Laravel project or use an existing one. You can create a new Laravel project using Composer by running the following command in your terminal:
composer create-project --prefer-dist laravel/laravel api-project
Next, navigate to your project directory and start the Laravel development server using the `php artisan serve` command. With your Laravel project up and running, you’re ready to start developing your RESTful API endpoints.
**Creating API Routes in Laravel**
In Laravel, routes are defined in the `routes/api.php` file for API-specific routes. Let’s create a simple API route that responds with a JSON message:
php
Route::get('/hello', function () {
return response()->json(['message' => 'Hello, World!']);
});
This route will respond with a JSON message when accessed via `GET /hello`.
**CRUD Operations with Laravel API Resources**
Laravel provides a convenient way to transform models and model collections into JSON using API Resources. Let’s create a basic API endpoint for CRUD (Create, Read, Update, Delete) operations on a hypothetical `Product` model:
1. Generate the `Product` model and its corresponding migration:
php artisan make:model Product -m
2. Define the migration fields and run the migration:
3. Create a corresponding API Resource for the `Product` model:
php artisan make:resource ProductResource
4. Implement CRUD operations in your `ProductController` using the `ProductResource`:
php
use App\Models\Product;
use App\Http\Resources\ProductResource;
public function index()
{
$products = Product::all();
return ProductResource::collection($products);
}
public function store(Request $request)
{
$product = Product::create($request->all());
return new ProductResource($product);
}
These are just a few examples of how Laravel simplifies RESTful API development. By following Laravel’s conventions and using its built-in features, you can create powerful APIs with minimal effort.
**Conclusion**
In this blog post, we’ve covered the basics of Laravel 11 RESTful API development, including setting up a Laravel project, defining API routes, and implementing CRUD operations with API Resources. Laravel’s elegant syntax and powerful features make it an ideal choice for building RESTful APIs that are well-structured, efficient, and scalable.
If you’re new to API development or looking to enhance your skills with Laravel, exploring RESTful API development in Laravel 11 is a great place to start. Happy coding!
Post Your Questions on our forum
Post a question on Forum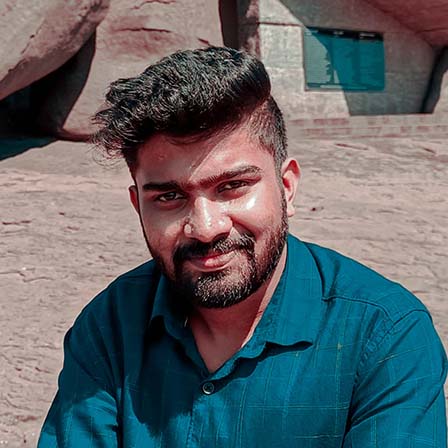
Share with your friends:
How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this […]
April 3, 2024
How to integrate Razorpay API in Laravel
Integrating payment gateways into web applications has become an essential part of e-commerce websites. In this tutorial, we will discuss […]
April 3, 2024
Laravel 11 Ajax CRUD Operation Tutorial Example
**Mastering CRUD Operations with Laravel 11 Ajax: A Comprehensive Tutorial** In the world of web development, interaction between the front-end […]
April 3, 2024
Login as Client in Laravel – Login with user id
**Unlock the Power of Laravel with Login as Client – Login with User ID** Laravel, the popular PHP framework, offers […]
April 3, 2024
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now