Laravel Database Seeder Example Tutorial
Title: A Comprehensive Guide to Laravel Database Seeder Example Tutorial
Are you looking to populate your Laravel application database with sample data quickly and efficiently? Database seeders in Laravel provide a convenient way to do just that! In this tutorial, we will walk you through the concept of Laravel database seeders and show you how to create and utilize them to seed your database with sample data.
**What are Laravel Database Seeders?**
Laravel database seeders allow you to insert sample or test data into your application’s database tables. This can be useful for various purposes, such as populating your database with initial data for testing, seeding demo environments, or creating fake data for development purposes.
**Creating a Seeder in Laravel**
To create a new seeder in Laravel, you can use the `make:seeder` Artisan command:
bash
php artisan make:seeder UsersTableSeeder
This command will generate a new seeder class in the `database/seeders` directory. In this example, we are creating a seeder named `UsersTableSeeder`.
**Writing Seeder Logic**
Open the generated seeder file, and you will find a `run()` method. Inside this method, you can define the logic to insert data into your database tables. You can use Laravel’s Eloquent models or the query builder to insert records.
Here is an example of populating a `users` table with sample data using the Eloquent model:
php
use App\Models\User;
use Illuminate\Database\Seeder;
class UsersTableSeeder extends Seeder
{
public function run()
{
User::create([
'name' => 'John Doe',
'email' => '[email protected]',
'password' => bcrypt('password123'),
]);
// Insert more sample data here
}
}
**Running Seeders**
To run the seeders and insert the sample data into your database, you can use the `db:seed` Artisan command:
bash
php artisan db:seed --class=UsersTableSeeder
This command will execute the `run()` method in the `UsersTableSeeder` class and populate the `users` table with the sample data.
**Seeders and Database Refresh**
Seeders can be particularly useful when refreshing the database during development or testing. You can combine seeders with the `migrate:refresh` command to reset and reseed the database:
bash
php artisan migrate:refresh --seed
This command will roll back all migrations, re-run them, and then reseed the database tables using the defined seeders.
**Conclusion**
In this tutorial, we have covered the concept of Laravel database seeders and demonstrated how to create, write, and run seeders to populate your database with sample data. Seeders are an essential tool for managing and seeding databases in Laravel applications, making the process of seeding data quick and efficient.
We hope this tutorial has been helpful in understanding Laravel database seeders and how you can leverage them in your projects. Happy seeding!
**References:**
– Laravel Documentation: https://laravel.com/docs/seeding
Post Your Questions on our forum
Post a question on Forum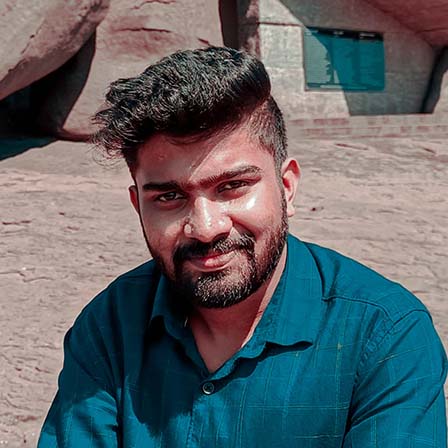
Share with your friends:
How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this […]
April 3, 2024
How to integrate Razorpay API in Laravel
Integrating payment gateways into web applications has become an essential part of e-commerce websites. In this tutorial, we will discuss […]
April 3, 2024
Laravel 11 Ajax CRUD Operation Tutorial Example
**Mastering CRUD Operations with Laravel 11 Ajax: A Comprehensive Tutorial** In the world of web development, interaction between the front-end […]
April 3, 2024
Login as Client in Laravel – Login with user id
**Unlock the Power of Laravel with Login as Client – Login with User ID** Laravel, the popular PHP framework, offers […]
April 3, 2024
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now