Laravel Multiple Image Upload Tutorial Example
**Laravel Multiple Image Upload Tutorial: Enhance Your Web Development Skills**
Are you looking to level up your Laravel skills and incorporate a feature that allows users to upload multiple images at once? Well, you’re in luck! In this tutorial, I will guide you through the process of implementing multiple image upload functionality in Laravel. By the end of this guide, you’ll have a solid understanding of how to handle multiple image uploads efficiently in your Laravel application.
**Setting Up the Project**
Before we dive into the code, make sure you have Laravel installed on your system. If not, you can refer to the official Laravel documentation for installation instructions.
To get started, create a new Laravel project by running the following command in your terminal:
bash
laravel new MultipleImageUpload
Next, navigate to the project directory:
bash
cd MultipleImageUpload
**Creating the Image Model and Migration**
Let’s create a model and migration file for the images. Run the following command to generate the model and migration:
bash
php artisan make:model Image -m
Head over to the newly created migration file located in the `database/migrations` directory. Open the migration file and define the schema for the images table. Here’s an example schema for the images table:
php
public function up()
{
Schema::create('images', function (Blueprint $table) {
$table->id();
$table->string('image_path');
$table->timestamps();
});
}
Don’t forget to run the migration to create the images table:
bash
php artisan migrate
**Creating the Image Upload Form**
Now, let’s create a form with the ability to upload multiple images. In your Blade view file, add the following form markup:
html
<form action="{{ route('upload.images') }}" method="post" enctype="multipart/form-data">
@csrf
<input type="file" name="images[]" multiple>
<button type="submit">Upload Images</button>
</form>
**Handling Image Upload in the Controller**
Next, we need to create a controller to handle the image upload process. Generate a new controller by running the following command:
bash
php artisan make:controller ImageController
In your `ImageController`, write a method to handle the image upload logic:
php
public function uploadImages(Request $request)
{
if($request->hasFile('images')) {
foreach ($request->file('images') as $image) {
$imageName = time().'.'.$image->getClientOriginalExtension();
$image->move(public_path('images'), $imageName);
Image::create(['image_path' => $imageName]);
}
}
return redirect()->back()->with('success', 'Images uploaded successfully.');
}
Don’t forget to import the necessary classes at the top of your controller:
php
use App\Models\Image;
use Illuminate\Http\Request;
**Routing**
Now, let’s define the route that points to the `uploadImages` method in the `ImageController`. Open your `web.php` routes file and add the following route definition:
php
Route::post('/upload/images', [ImageController::class, 'uploadImages'])->name('upload.images');
**Displaying Uploaded Images**
To display the uploaded images, you can fetch them from the database and render them in your Blade view file. Make sure to import the `Image` model at the top of your controller:
php
use App\Models\Image;
In your view file, you can loop through the images and display them using HTML `` tags.
html
@foreach($images as $image)
<img src="{{ asset('images/'.$image->image_path) }}" alt="Image">
@endforeach
**Conclusion**
Congratulations! You’ve successfully implemented multiple image upload functionality in your Laravel application. This feature can enhance the user experience and make your application more dynamic and engaging. Feel free to customize and extend this functionality to suit your specific needs. Happy coding!
Post Your Questions on our forum
Post a question on Forum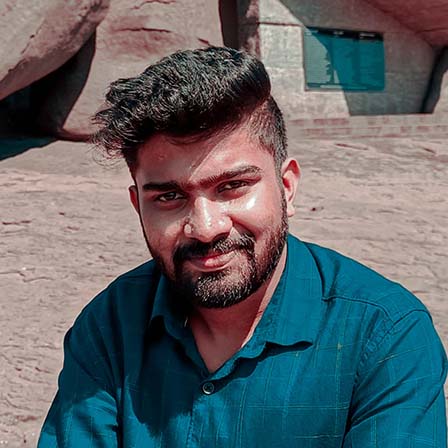
Share with your friends:
How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this […]
April 3, 2024
How to integrate Razorpay API in Laravel
Integrating payment gateways into web applications has become an essential part of e-commerce websites. In this tutorial, we will discuss […]
April 3, 2024
Laravel 11 Ajax CRUD Operation Tutorial Example
**Mastering CRUD Operations with Laravel 11 Ajax: A Comprehensive Tutorial** In the world of web development, interaction between the front-end […]
April 3, 2024
Login as Client in Laravel – Login with user id
**Unlock the Power of Laravel with Login as Client – Login with User ID** Laravel, the popular PHP framework, offers […]
April 3, 2024
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now