Routing 101 in Laravel
Title: Mastering Routing 101 in Laravel: A Comprehensive Guide
Routing is a fundamental concept in web development that determines how an application responds to a client request. In Laravel, a powerful PHP framework, routing plays a crucial role in defining the structure of your application’s URLs and mapping them to the appropriate controllers and actions. In this blog post, we will delve into Routing 101 in Laravel to help you master this essential concept.
**Understanding Laravel Routing**
In Laravel, routes are defined in the `routes/web.php` file by using the `Route` facade. Routes can be defined using the `get`, `post`, `put`, `patch`, `delete`, and `any` methods. The most commonly used methods are `get` and `post`, which correspond to the HTTP GET and POST requests, respectively.
php
Route::get('/about', function () {
return "Welcome to the About page!";
});
Route::post('/contact', function () {
return "Contact form submitted successfully!";
});
In the above code snippets, we have defined routes for the `/about` and `/contact` URLs using the `get` and `post` methods, respectively. When a user navigates to the `/about` URL, they will see the “Welcome to the About page!” message, and when they submit a form to the `/contact` URL, they will receive the “Contact form submitted successfully!” message.
**Route Parameters and Named Routes**
Laravel allows you to define routes with parameters to capture dynamic segments of the URL. Parameters are enclosed in curly braces `{}` and are passed as arguments to the route callback function.
php
Route::get('/user/{id}', function ($id) {
return "User ID: " . $id;
})->name('user.profile');
In the above example, the `{id}` parameter captures the user’s ID from the URL and passes it to the route callback function. You can also assign a name to a route using the `name` method, making it easier to generate URLs using route names rather than URLs.
**Route Groups and Middleware**
Route groups allow you to apply common attributes, such as middleware, namespaces, and prefixes, to a group of routes. Middleware is a mechanism for filtering HTTP requests before they reach your application’s routes. You can apply middleware to a single route or a group of routes to perform authentication, authorization, and other tasks.
php
Route::middleware('auth')->group(function () {
Route::get('/dashboard', function () {
return "Welcome to the dashboard!";
});
Route::get('/settings', function () {
return "User settings page";
});
});
In the above example, we have applied the `auth` middleware to the route group, ensuring that only authenticated users can access the `/dashboard` and `/settings` routes.
**Route Caching and Route Model Binding**
Laravel provides route caching to improve the performance of your application by storing compiled route definitions in a file. You can generate a route cache file using the `route:cache` Artisan command, which speeds up the process of registering routes when the application is bootstrapped.
Route model binding is a convenient feature in Laravel that automatically resolves Eloquent models passed in route parameters. By type-hinting model instances in route definitions, Laravel will fetch the corresponding model by its primary key and pass it to the route callback function.
php
Route::get('/user/{user}', function (App\Models\User $user) {
return $user;
});
In the above example, the `{user}` parameter is resolved to an instance of the `User` model based on the user’s ID.
**Conclusion**
Mastering routing in Laravel is essential for building robust and scalable web applications. By understanding the concepts of route definition, parameters, groups, middleware, caching, and model binding, you can create elegant and efficient routing structures in your Laravel projects. Experiment with different route configurations and explore advanced routing features to enhance the functionality and usability of your applications. Happy routing!
Post Your Questions on our forum
Post a question on Forum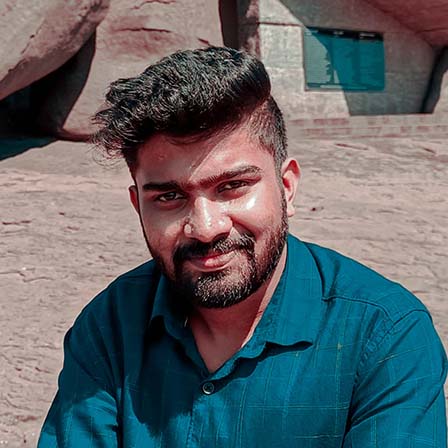
Share with your friends:
How to integrate Paypal API in Laravel
Are you looking to integrate Paypal API in your Laravel project for seamless payment processing? Look no further! In this […]
April 3, 2024
How to integrate Razorpay API in Laravel
Integrating payment gateways into web applications has become an essential part of e-commerce websites. In this tutorial, we will discuss […]
April 3, 2024
Laravel 11 Ajax CRUD Operation Tutorial Example
**Mastering CRUD Operations with Laravel 11 Ajax: A Comprehensive Tutorial** In the world of web development, interaction between the front-end […]
April 3, 2024
Login as Client in Laravel – Login with user id
**Unlock the Power of Laravel with Login as Client – Login with User ID** Laravel, the popular PHP framework, offers […]
April 3, 2024
Digital Marketing Toolkit
Get Free Access to Digital Marketing Toolkit. You can use all our tools without any limits
Get Free Access Now